Connectionless sockets do not establish a connection over which
data is transferred. Instead, the server application specifies its name where
a client can send requests.
Connectionless sockets use User Datagram Protocol (UDP) instead
of TCP/IP.
The
following figure illustrates the client/server relationship from the socket
APIs used in the code examples for a connectionless socket design.
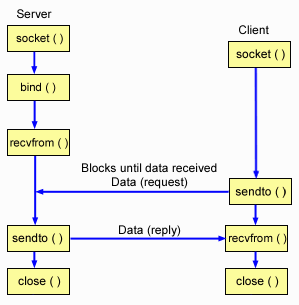
Socket flow of events: Connectionless server
The
following sequence of the socket calls provide a description of the figure
and the following example programs. It also describes the relationship
between the server and client application in a connectionless design. Each
set of flows contain links to usage notes on specific APIs. If you need more
details on the use of a particular API, you can use these links. The first
example uses the following sequence of function calls:
- The socket() function returns a socket descriptor representing
an endpoint. The statement also identifies that the INET (Internet Protocol)
address family with the UDP transport (SOCK_DGRAM) is used for this socket.
- After the socket descriptor is created, a bind() function
gets a unique name for the socket. In this example, the user sets the s_addr
to zero, which means that the UDP port of 3555 is bound to all IPv4 addresses
on the system.
- The server uses the recvfrom() function to receive
that data. The recvfrom() function waits indefinitely for
data to arrive.
- The sendto() function echoes the data back to the client.
- The close() function ends any open socket descriptors.
Socket flow of events: Connectionless client
The
second example uses the following sequence of function calls:
- The socket() function returns a socket descriptor representing
an endpoint. The statement also identifies that the INET (Internet Protocol)
address family with the UDP transport (SOCK_DGRAM) is used for this socket.
- In the client example program, if the server string that was passed into
the inet_addr() function was not a dotted decimal IP address, then it is assumed
to be the hostname of the server. In that case, use the gethostbyname() function
to retrieve the IP address of the server.
- Use the sendto() function to send the data to the server.
- Use the recvfrom() function to receive the data back
from the server.
- The close() function ends any open socket descriptors.